BACK TO THE MINE
SimpleMiner is a simplified Minecraft clone that features a voxel-based world, light propogation, and procedurally generated environments.
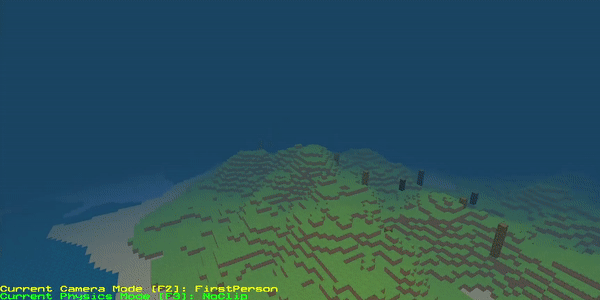
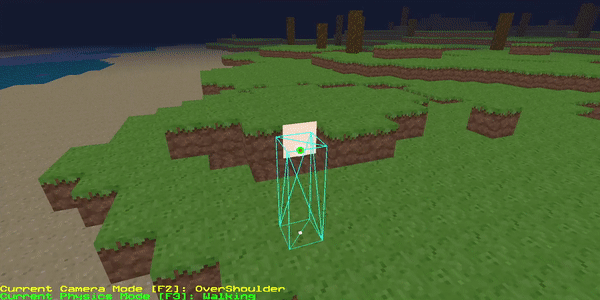
void World::ProcessNextDirtyLightBlock() { BlockIterator bIter = m_dirtyLightQueue.front(); // Compute Lighting int idealIndoorLight = GetIdealIndoorLight( bIter ); int idealOutdoorLight = GetIdealOutdoorLight( bIter ); if( bIter.GetBlock()->GetIndoorLightExposure() != idealIndoorLight || bIter.GetBlock()->GetOutdoorLightExposure() != idealOutdoorLight ) { bIter.GetBlock()->SetIndoorLightExposure( idealIndoorLight ); bIter.GetBlock()->SetOutdoorLightExposure( idealOutdoorLight ); bIter.m_chunk->SetChunkAsDirty(); // Add Neighbors to Queue MarkLightingDirtyIfNotOpaque( bIter.GetNorthNeighbor() ); MarkLightingDirtyIfNotOpaque( bIter.GetEastNeighbor() ); MarkLightingDirtyIfNotOpaque( bIter.GetSouthNeighbor() ); MarkLightingDirtyIfNotOpaque( bIter.GetWestNeighbor() ); MarkLightingDirtyIfNotOpaque( bIter.GetTopNeighbor() ); MarkLightingDirtyIfNotOpaque( bIter.GetBottomNeighbor() ); } bIter.GetBlock()->SetIsBlockLightDirty( false ); m_dirtyLightQueue.pop_front(); }
Post-Mortem
There were many moments in the development of SimpleMiner where I had to seriously consider the performance of the game and how each new system affected it, from procedurally generating the world chunk-by-chunk, to saving and loading chunk data, to updating the lighting when a block was added or removed. One simple error could tank the game’s framerate. Since performance affected my ability to create and debug new features, efficiency was key. This project was also a first look into many topics in the context of game development, like noise-based generation and multithreading.